Connect a Universal Profile
There are several methods to connect to a Universal Profile, each catering to different developer requirements and scenarios. Below, we detail the most common approaches and explain why a developer might prefer one over the others.
Connecting to the Universal Profile Browser Extension will trigger the following connection screen:
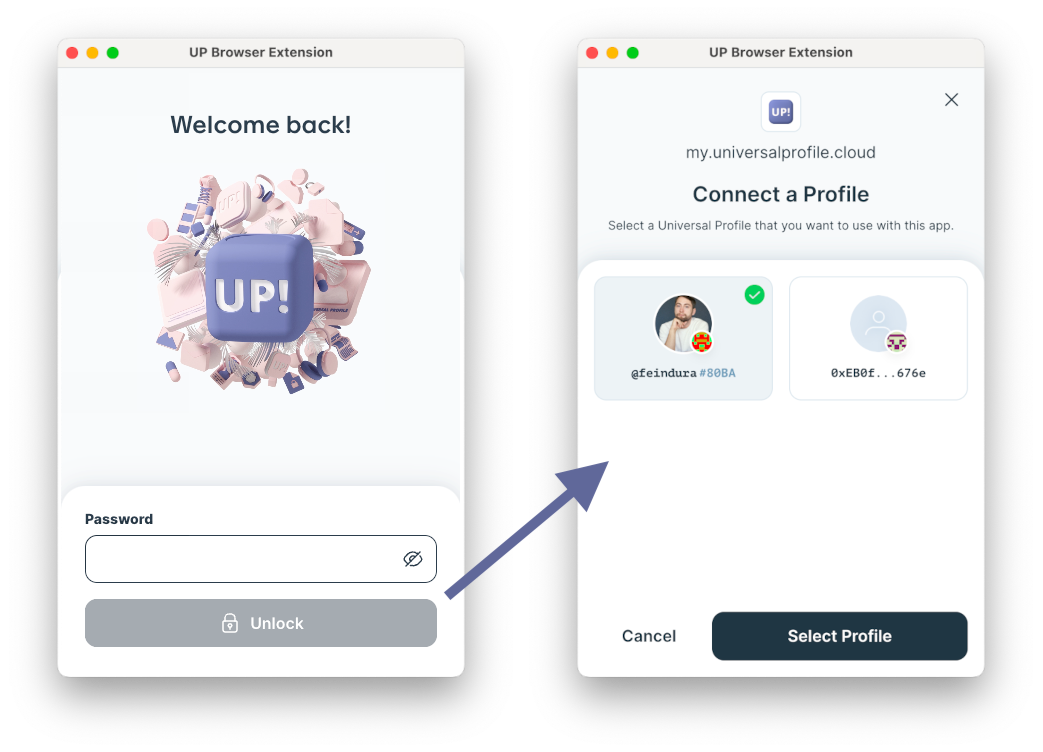
The Universal Profile Extension returns the address of the connected Universal Profile. Making transactions is the same as with any wallet, you just use the profile address as a from
in your transactions.
Connect with EIP-6963â
Using EIP-6963 Provider Discovery is the latest industry standardization, solving previous connectivity issues when having multiple wallet extensions installed at the same time.
You can listen to eip6963:announceProvider
events following the EIP-6963: Multi Injected Provider standardization to facilitate a more versatile connection to multiple wallet extensions. This method is beneficial for developers who require the ability to maintain low-level control over how different extensions are targeted and managed within their dApp.
let providers = [];
window.addEventListener("eip6963:announceProvider", (event) => {
providers.push(event.detail);
});
// Request installed providers
window.dispatchEvent(new Event("eip6963:requestProvider"));
...
// pick a provider to instantiate (providers[n].info)
const provider = new Web3(providers[0].provider);
const accounts = await provider.eth.requestAccounts();
console.log('Connected with', accounts[0]);
If you want to implement Injected Provider Discovery you can visit our Example EIP-6963 Test dApp.
Multi-Provider Librariesâ
You can use third-party libraries like Web3Modal from Wallet Connect or Web3-Onboard from Blocknative. Both libraries come with built-in UI elements and allow you to connect to various wallet extensions with ease. This method is beneficial if you want to support multiple extensions without them all supporting EIP-6963.
If you want to implement a Multi-Provider Library, you can follow our Multi-Provider Connections Guide or check out the implementation within our dApp Boilerplate.
Use Provider Injectionâ
You can use the window.lukso
object, tailored for a direct integration with the UP Browser Extension. This approach allows developers to engage directly with the UP Browser Extension without the need to consider compatibility with other extensions.
- ethers
- web3
npm install ethers
npm install web3
- ethers
- web3
import { ethers } from 'ethers';
const provider = new ethers.BrowserProvider(window.lukso);
const accounts = await provider.send('eth_requestAccounts', []);
console.log('Connected with', accounts[0]);
import Web3 from 'web3';
const provider = new Web3(window.lukso);
const accounts = await provider.eth.requestAccounts();
console.log('Connected with', accounts[0]);
Alternatively to the window.lukso
, the equivalent window.ethereum
object can be called within supported browsers, just like other Ethereum wallets. Both follow the EIP-1193 Ethereum Provider JavaScript API. You can use a simple fallback to allow regular wallet connections, if the Universal Profile Browser Extension is not installed:
- ethers
- web3
const providerObject = window.lukso || window.ethereum;
const provider = new ethers.BrowserProvider(providerObject);
const providerObject = window.lukso || window.ethereum;
const provider = new Web3(providerObject);