Interact with other contracts
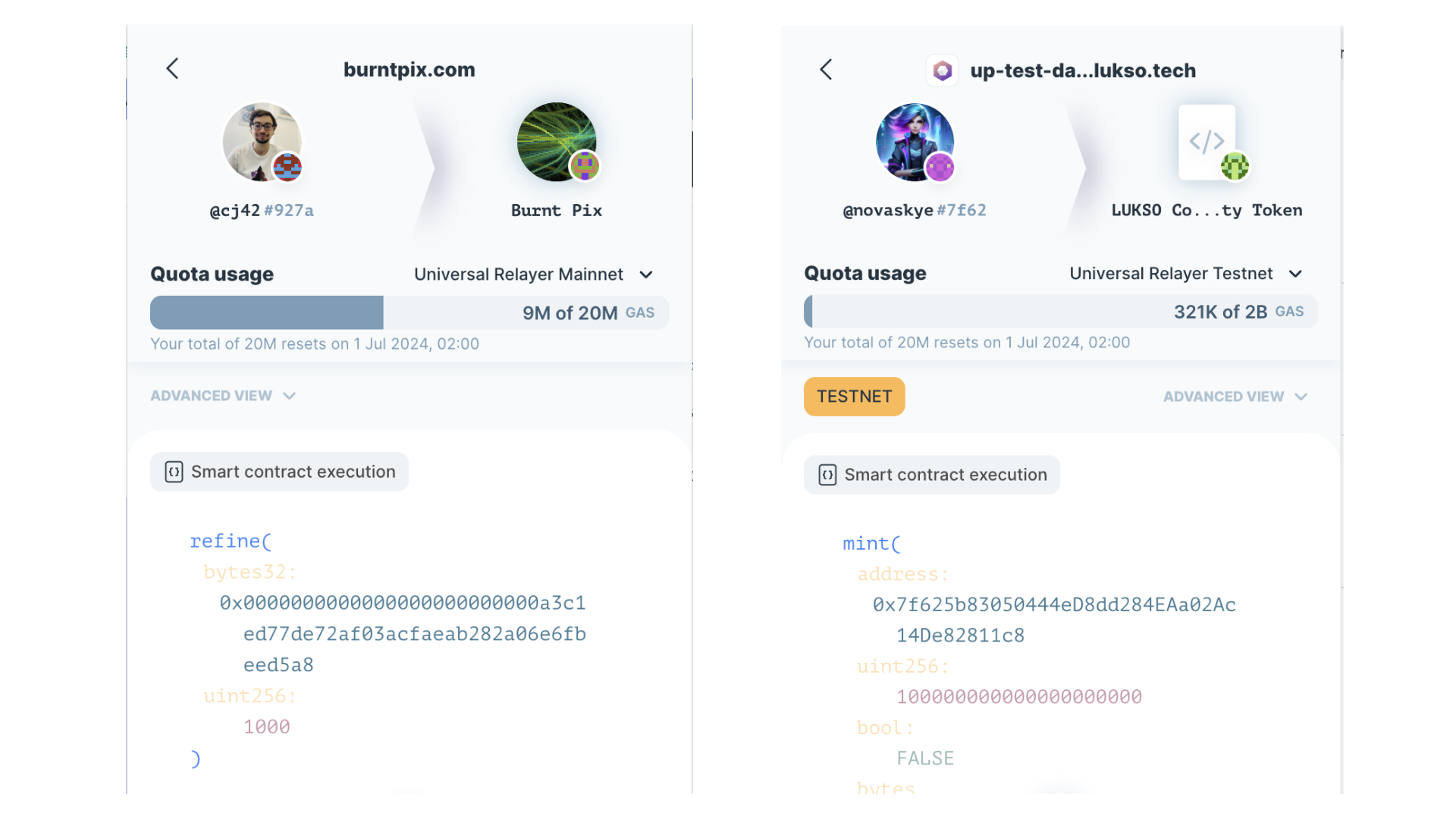
Examples of interacting with contracts, like minting tokens or refining burntpix.
In this guide, you will learn how to use your Universal Profile to interact with any other smart contract.
You will see from the examples below that there is no difference with writing the code for interacting with regular EOA-based wallet vs using Universal Profile. The code is the same! Simply:
- create an instance of the contract you want to interact with.
- call the function you want on this contract.
- use the π address as
{ from: "0x..." }
in the transaction options.
Setupβ
To complete this guide, we will need some initial constants values and install some dependencies
- the address of the contract we want to interact with.
- the ABI of the contract we want to interact with.
- Either
web3.js
orethers.js
@lukso/lsp-smart-contracts
- ethers
- web3
npm install ethers @lukso/lsp-smart-contracts
npm install web3 @lukso/lsp-smart-contracts
Interactions Examplesβ
Below you will find some examples to perform the following:
- Mint a free NFT and see it appear in your wallet on universalprofile.cloud.
- Execute a swap on UniversalSwap.io
- Refine a BurntPix
Example 1 - Mint some Tokensβ
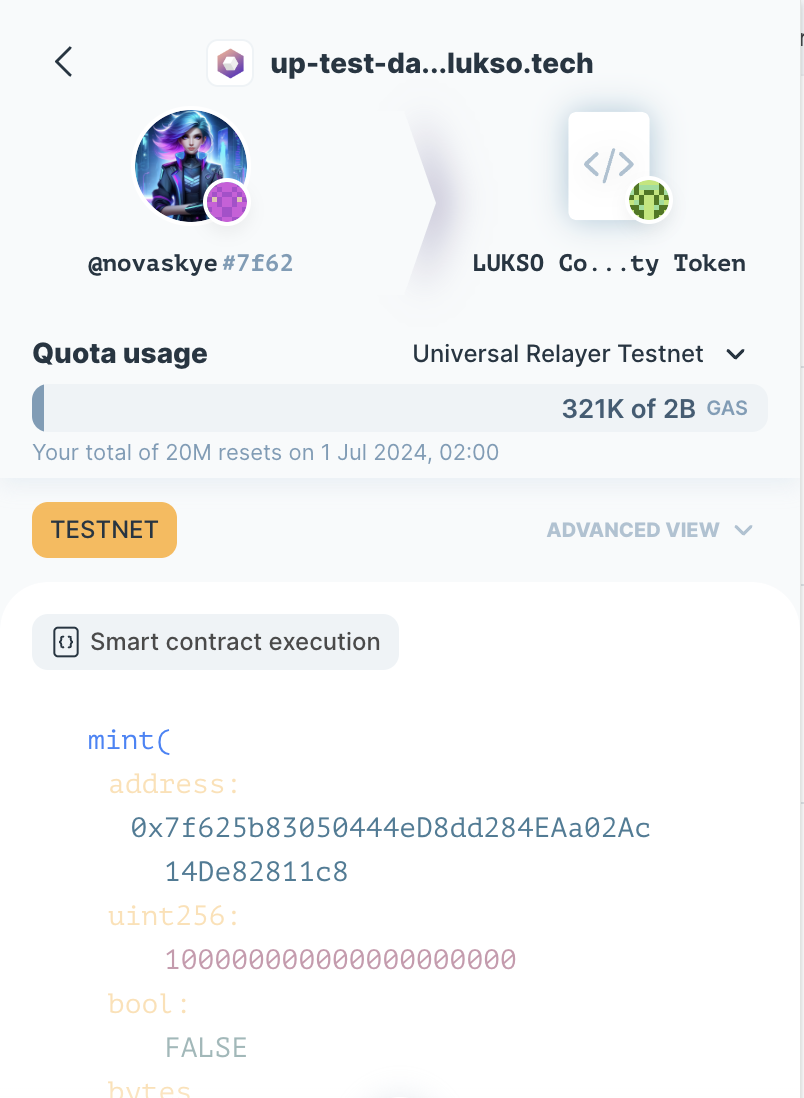
- ethers
- web3
mintTokens.ts
import { ethers } from 'ethers';
import LSP7Mintable from '@lukso/lsp-smart-contracts/artifacts/LSP7Mintable.json';
const TOKEN_CONTRACT_ADDRESS = '0x...';
await ethers.provider.send('eth_requestAccounts', []);
const universalProfile = await ethers.getSigner();
const myToken = new ethers.Contract(TOKEN_CONTRACT_ADDRESS, LSP7Mintable.abi);
const mintTxn = await myToken.mint(
universalProfile.address, // recipient address
ethers.parseUnits('100', 'ether'), // token amount (mint 100 tokens)
true, // force parameter
'0x', // additional data
{
from: universalProfile,
},
);
console.log(mintTxn);
const balance = await myToken.balanceOf(signer.address);
console.log('π¦ Balance: ', balance.toString());
mintTokens.ts
import Web3 from 'web3';
import LSP7Mintable from '@lukso/lsp-smart-contracts/artifacts/LSP7Mintable.json';
const web3 = new Web3(window.lukso);
await web3.eth.requestAccounts();
const accounts = await web3.eth.getAccounts();
const universalProfile = accounts[0];
const myToken = new web3.eth.Contract(
LSP7Mintable.abi,
'0x6E872Eb9942db637cd82ad7C33C2206eACB81181', // Token contract address
);
const mintTxn = await myToken.methods
.mint(
universalProfile, // recipient address
web3.utils.toWei('100', 'ether'), // token amount (mint 100 tokens)
true, // force parameter
'0x', // additional data
)
.send({ from: universalProfile });
console.log(mintTxn);
const balance = await myToken.methods.balanceOf(accounts[0]);
console.log('π¦ Balance: ', balance.toString());
Example 2 - Refine a BurntPixβ
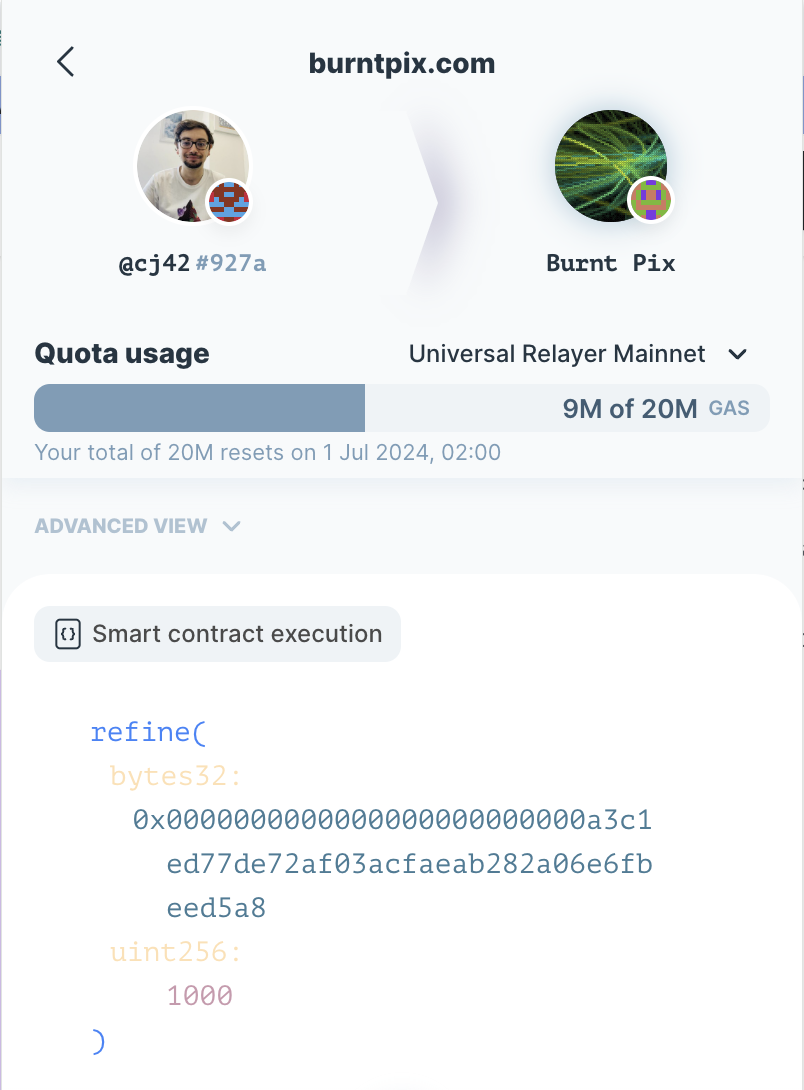
- ethers
- web3
refineBurntPix.ts
import { ethers } from 'ethers';
// Constants:
// - BurntPix Registry contract to interact with
const BURNT_PIX_REGISTRY_ADDRESS = "0x3983151E0442906000DAb83c8b1cF3f2D2535F82";
// - bytes32 ID of the BurntPix to refine
const BURNT_PIX_ID "0x0000000000000000000000000a3c1ed77de72af03acfaeab282a06e6fbeed5a8";
// 1. Connect to UP Browser Extension
const provider = new ethers.BrowserProvider(window.lukso);
const accounts = await provider.send('eth_requestAccounts', []);
const universalProfile = accounts[0];
// 2. Create an instance of the BurntPix Registry contract
const burntPixRegistry = new ethers.Contract(
BURNT_PIX_REGISTRY_ADDRESS,
["function refine(bytes32 tokenId, uint256 iterations) external"]
);
// Perform 500 iteration to refine a specific Burnt Pix
await contract.refine(BURNT_PIX_ID, "500", {
from: universalProfile,
gasPrice: ethers.formatUnits("1", 'gwei'),
gasLimit: 15_000_000,
});
refineBurntPix.ts
import Web3 from 'web3';
// 1. Connect to UP Browser Extension
const provider = new Web3(window.lukso);
const accounts = await provider.eth.requestAccounts();
const universalProfile = accounts[0];
// 2. Create an instance of the BurntPix Registry contract
const burntPixRegistry = new web3.eth.Contract(BURNT_PIX_REGISTRY_ADDRESS, [
refineFunctionABI,
]);
// Perform 500 iteration to refine a specific Burnt Pix
await contract.methods.refine(BURNT_PIX_ID, '500').send({
from: universalProfile,
gasPrice: web3.utils.fromWei('1000000000', 'gwei'),
gasLimit: 15_000_000,
});